A often unneeded, yet sometimes required feature, put into a library: Modifying registry elements at runtime. Not much explanation is needed for this library, other than if used improperly it can cause errors.
How to use:
void Initialize() {
...
//Note: do NOT use Registry.register(...) on the custom assets!
RegistryEditEvent.EVENT.register(manipulator -> {
// For native Minecraft elements (Item/Block)
var myCustomItem1 = new Item(new Item.Settings());
manipulator.Redirect(Registries.ITEM, Items.STICK, myCustomItem1);
// ++ Items.STICK = myCustomItem1
// -- Items.STICK = EXISTING_ITEM
// other elements (Any, so long as the field holding it is static final)
var myCustomItem2 = new Item(new Item.Settings());
manipulator.Redirect(MyItems.class, Registries.ITEM, MyItems.CUSTOM_ITEM, myCustomItem2);
// ++ MyItems.CUSTOM_ITEM = myCustomItem2
// -- MyItems.CUSTOM_ITEM = EXISTING_ITEM
// For removing elements entirely (VERY DANGEROUS UNLESS YOU KNOW WHAT YOU ARE DOING!)
manipulator.Unregister(Registries.ITEM, Items.ENDER_PEARL);
// If you are removing a block, ensure you replace the BlockItem associated with it!
manipulator.Unregister(Registries.BLOCK, Blocks.COBBLESTONE);
manipulator.Redirect(Registries.Item, Items.COBBLESTONE, new Item(new Item.Settings()));
});
...
}
Assuming you are overriding an item in the Items / Blocks class (ie, where Items.STICK would be located) the registry edit will apply to that variable as well. If you need to modify an element that isn't in the Items / Blocks class, you can provide your holder class.
Featured versions
See allProject members
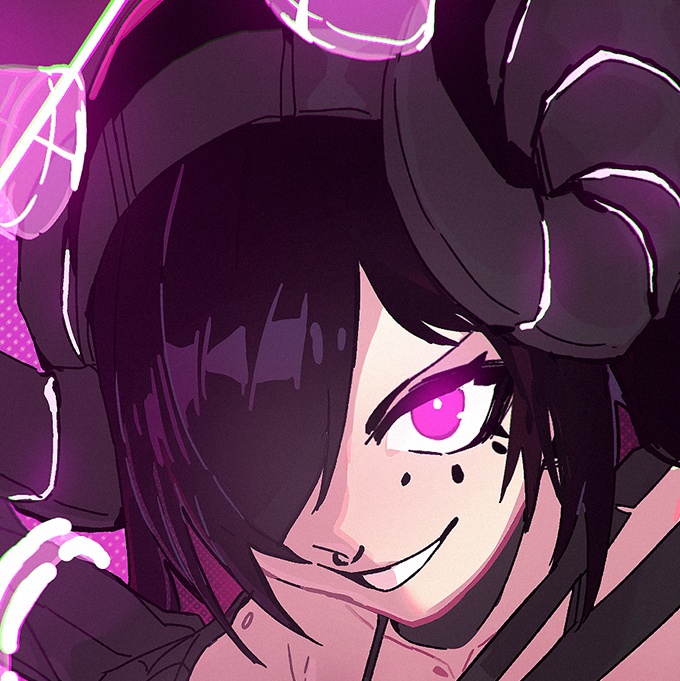
Feintha
Member
Technical information
License
MIT
Client side
optional
Server side
optional
Project ID