Compatibility
Minecraft: Java Edition
Platforms
Supported environments
75% of ad revenue goes to creators
Support creators and Modrinth ad-free with Modrinth+Creators
Details
Unruled API
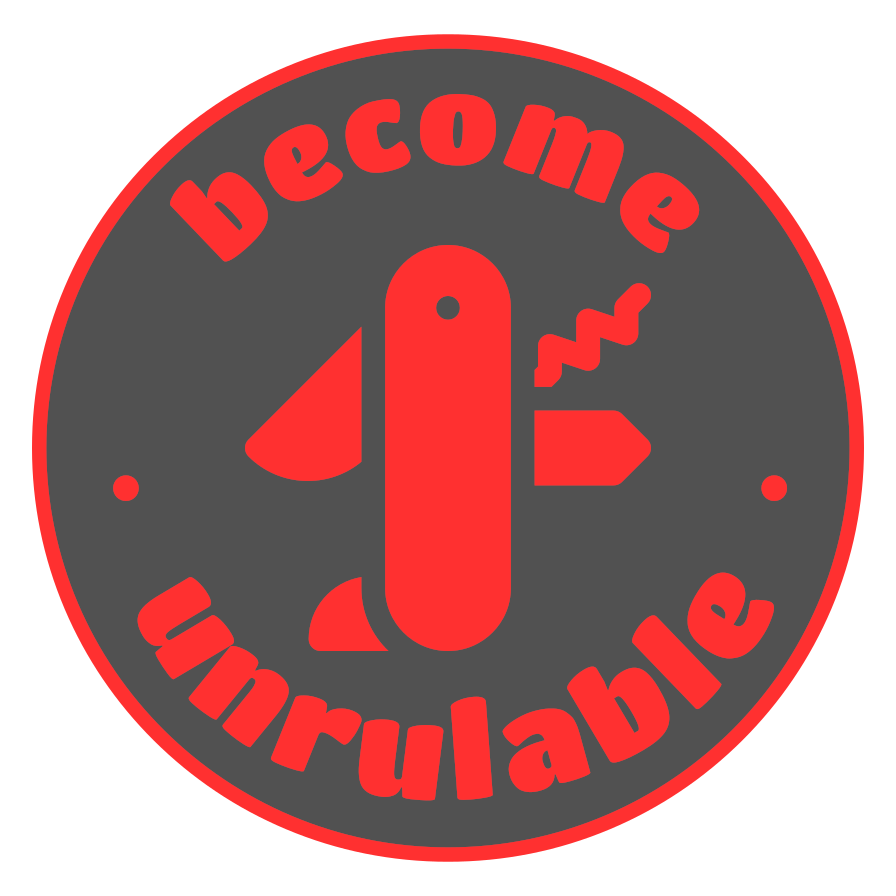
Allows to create new form of gamerules, beyond the restrictive vanilla integers and booleans.
This mod enables developers to easily create new floating, long, double, string, text, enum-driven,
entity selector and even registry-entry gamerules, using their respective builders,
all available from the mc.recraftors.unruled_api.UnruledApi
class's static methods.
Additionally, this mod also adds per-dimension gamerule overrides! These are applied in their strict dimension (provided they are queried correctly). See gamerules overrides for more details about it.
Gamerules registration
Example:
import mc.recraftors.unruled_api.UnruledApi;
public class MyClass {
private static final int STRING_RULE_MAX_LENGTH = 24;
public void gamerulesRegistration() {
var myFloatingRule = UnruledApi.floatRuleBuilder(1.5) // takes the initial value as argument
.register("my_gamerule", category); // gamerule registration always takes the gamerule's name and category.
// The former determines its name and translations, the latter where to place it in the world creation screen.
var myStringRule = new StringRule.Builder("initial value", STRING_RULE_MAX_LENGTH)
// using the UnruledApi methods or directly creating the builder has the same result, and arguments are the same.
.register("my_other_gamerule", category);
}
}
Additionally, quick creation and registration methods have been added for your convenience
import mc.reraftors.unruled_api.UnruledApi;
public static void quickGamerulesRegistration() {
var myLongRule = UnruledApi.registerLongRule("my_long_rule", category, 25L);
// Quick registration methods always follow the same parameter order: name, category, initial value, gamerule-type specific arguments
// Except for enum rules, for which the enum class goes before the initial value
var myEnumRule = UnruledApi.registerEnumRule("my_enum_rule", category, MyEnum.class, MyEnum.FIRST);
}
enum MyEnum {
FIRST,
SECOND,
THIRD
}
For consistency, methods have also been created to allow quickly register vanilla-type gamerules.
import mc.recraftors.unruled_api.UnruledApi;
public static void registerBasicRules() {
UnruledApi.registerInt("my_int_rule", category, 5);
UnruledApi.registerBoolean("my_bool_rule", category, false);
}
You can also retrieve their values using the same class's methods
public long getMyLongGamerules(ServerWorld dimension) { // ServerWorld is a Yarn mapping class. Might be Level or ServerLevel with Mojmap
return UnruledApi.getLong(dimension.getGameRules(), myLongGamerule);
}
public Block getMyBlockRule(ServerWorld dimension) {
// querying a registry entry rule returns the registry entry's very value, as it can only be set if it exists in the registry
return UnruledApi.getRegistryEntry(dimension.getGameRules(), myBlockRegistryEntryRule);
}
Gamerules Overrides
Gamerules overrides are set per dimension. They allow players to set specific rules to specific values
in the wanted dimension, all via the gamerule-override
command.
This command has many uses:
gamerule-override
alone and with no arguments allows you to get a list of existing overrides in the current dimension.gamerule-override <dimension id>
allows you to get a list of existing overrides in the specified dimension.gamerule-override get <gamerule name>
allows you to query a potential override for the specified gamerule in the current dimension.gamerule-override set <gamerule name> <value>
allows you to set an override for the specified gamerule to the given value in the current dimension.gamerule-override unset <gamerule name>
allows you to remove a set override for the specified gamerule in the current dimension.gamerule-override in <dimension id> get <gamerule name>
allows you to query a potential override for the specific gamerule in the given dimension.gamerule-override in <dimension id> set <gamerule name> <value>
allows you to set an override for the specified gamerule to the given value in the provided dimension.gamerule-override in <dimension id> unset <gamerule name>
allows you to remove a set override for the specified gamerule in the provided dimension.
Gamerule overrides are stored in the dimension's data folder, as the gamerules.dat
file.
Removing it while the server is offline/world is not being played will simply remove all overrides for the dimension.
How to use in your project
You can implement this mod in your project using the Modrinth maven. Don't hesitate to read the official documentation.
Add the Modrinth maven repository
repositories {
maven {
name = "Modrinth"
url = "https://api.modrinth.com/maven"
}
}
Import the mod
loom example:
dependencies {
// using modApi allows your project's dependents to also import dependencies by default
modApi "maven.modrinth:unruled-api:${project.unruled_version}"
}
(neo)forgeGradle example:
dependencies {
implementation "maven.modrinth:unruled-api:${project.unruled_version}"
}
For copyright reasons, we require you to not include (JiJ, jar-in-a-jar, shadow, etc) this mod inside of your own. Thank you for your comprehension.